In this blog post, we will be exploring the Django database, a popular Python web framework that makes it easy to build and maintain web applications. Working with databases is an essential aspect of many web applications, as it allows you to store and retrieve data that is used by your application.
Django provides a range of tools and features for working with databases, making it easy for developers to build applications that can store, retrieve, and manipulate data in a variety of ways. Whether you are building a simple application that stores user data or a more complex application that requires sophisticated database functionality, Django has you covered.
In the following sections, we will take a closer look at how to set up and work with databases in Django, as well as some of the advanced features that are available. So, let’s get started!
Django database settings
Django supports a wide range of database engines, including SQLite, PostgreSQL, and MySQL. You can choose the database engine that best fits your needs, depending on the complexity and scale of your application.
To set up a django database, you will need to specify the database engine and credentials in the Django settings file. This file is located in the “settings” directory of your Django project and is used to configure various aspects of your application, including the database settings.
To specify the database engine, you will need to set the “ENGINE” setting to the appropriate value. For example, to use SQLite, you would set “ENGINE” to “django.db.backends.sqlite3”. You will also need to set the “NAME” setting to the name of the database file. For example:
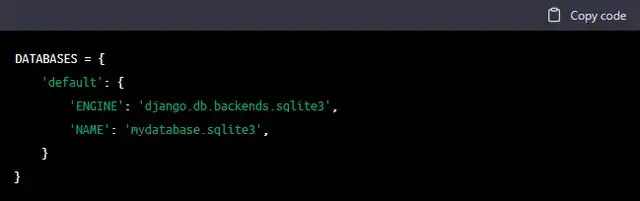
DATABASES = {
‘default’: {
‘ENGINE’: ‘django.db.backends.sqlite3’,
‘NAME’: ‘mydatabase.sqlite3’,
}
}
For other database engines, such as PostgreSQL or MySQL, you will need to provide additional settings, such as the hostname, username, and password. Consult the Django documentation for more information on configuring different database engines.
Read Also: SQL Server Data Types : 4 Best Practices for Choosing It
Once you have configured your database, you can use django database migration system to make changes to your database schema over time. This is useful when you need to add or remove fields from your models, or make other changes to the structure of your database. Django’s migration system makes it easy to manage these changes and ensure that your database remains in sync with your Django models.
Working with models
In Django, models describe data in the database and are defined in Python code. A model is a class that defines the fields and behaviors of the data you desire to store and any connections between various pieces of data.
To describe a Django model, you will require to make a Python class that subclasses django.db.models.Model and defines a series of class variables representing your model’s fields. For example:
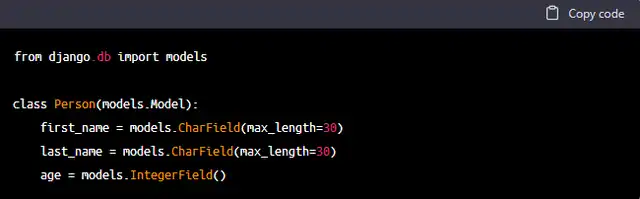
from django.db import models
class Person(models.Model):
first_name = models.CharField(max_length=30)
last_name = models.CharField(max_length=30)
age = models.IntegerField()
In this example, we have defined a model called “Person” with three fields: “first_name”, “last_name”, and “age.” Each field is represented by a class variable, an instance of a Django field class (e.g., CharField, IntegerField). The field class defines the type of data the field will store (e.g., text, integers) and any additional options (e.g., the maximum length for a CharField).
Django models can also define relationships between different models in complement to fields. It is useful when storing data associated with other data in your database. For example, you might have a “Person” model related to an “Address” model, which stores data about a person’s address. To define a relationship in django database, you can use Django’s ForeignKey field:
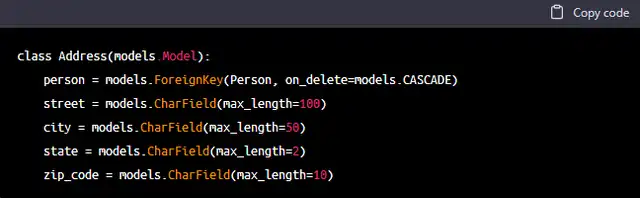
class Address(models.Model):
person = models.ForeignKey(Person, on_delete=models.CASCADE)
street = models.CharField(max_length=100)
city = models.CharField(max_length=50)
state = models.CharField(max_length=2)
zip_code = models.CharField(max_length=10)
In this model, the “Address” model has a ForeignKey field called “person” that refers to a “Person” instance. This establishes a one-to-many relationship between the “Person” and “Address” models, meaning that each person can have multiple addresses, but each address can only belong to one person.
Once you have defined your models, It provides a high-level API for querying the database and performing CRUD (create, read, update, delete) operations. This API is available through the Django ORM (Object-Relational Mapper), which authorizes you to interact with your database using Python code rather than SQL queries.
For example, you can use the .create() method to make a new record in the database, the .get() method to retrieve a specific record, and the .save() method to update a current record.
For example:.
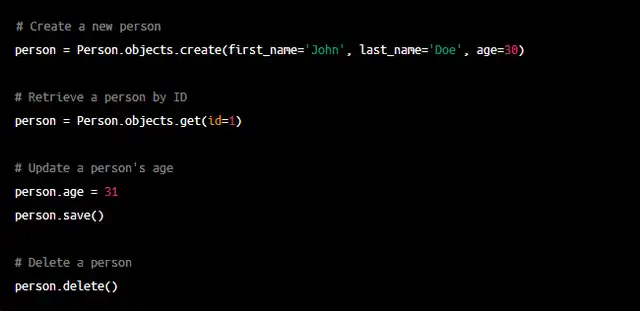
# Create a new person
person = Person.objects.create(first_name=’John’, last_name=’Doe’, age=30)
# Retrieve a person by ID
person = Person.objects.get(id=1)
# Update a person’s age
person.age = 31
person.save()
# Delete a person
person.delete()
Advanced database features
It provides several advanced database features that can improve your application’s performance and reliability. These features include:
Transactions: It supports database transactions, which allow you to group multiple database operations into a single atomic unit of work. Transactions ensure that your database remains consistent even if an error occurs during one of the functions. To use transactions in Django, you can employ the atomic decorator or context manager, which will automatically handle the transaction’s beginning and end.
Custom database managers: It lets you define custom managers for your models. It delivers a way to customize the default query set and add custom plans for operating with your model’s data. It can be useful for creating reusable query sets or adding convenience methods that make it easier to work with your data.
Database routing: The database routing feature lets you select other databases for other models or groups of models. It can be valuable when you include a complex application that requires multiple databases or when you need to scale your application by separating different data types into different databases.
To use these advanced database features in your Django application, you must modify your models and settings to enable them. Consult the django database documentation for more details on operating these features in your application.
Conclusion:
In conclusion, django database provides a range of tools and features for working with databases, making it easy to build and maintain web applications that require data storage and retrieval. Whether you are building a simple application that stores user data or a more complex application that requires sophisticated database functionality, Django has you covered.
With django database, you can set up a database and define models to represent your data, using a variety of field types and relationships to create a flexible and powerful data model. You can then use Django’s ORM to perform CRUD operations on your data, as well as advanced features like transactions and custom database managers to improve the performance and reliability of your application.
If you are new to Django and databases, we encourage you to try using these features in your own projects and to explore the Django documentation for more information. Working with databases in Django can be a rewarding and powerful experience, and we hope this blog post has helped to give you a better understanding of how it all works. Happy coding!
Add Comment