Are you looking to create a Python Rest Server? Have you heard of Flask and Gunicorn? These two awesome tools can help create a Python Rest Server incredibly easily! This blog post will walk you through exactly what a Python Rest Server is and how it works.
We also provide instructions on putting Flask and Gunicorn together to create your own Python Rest Server!
What is a Python Rest Server?
A Python Rest Server is a web application that allows you to manage client requests and responses.
A Python Rest Server can be used to create a secure REST API.
A Python Rest Server can be used for synchronous or asynchronous operations.
What are the benefits of using a REST Server?
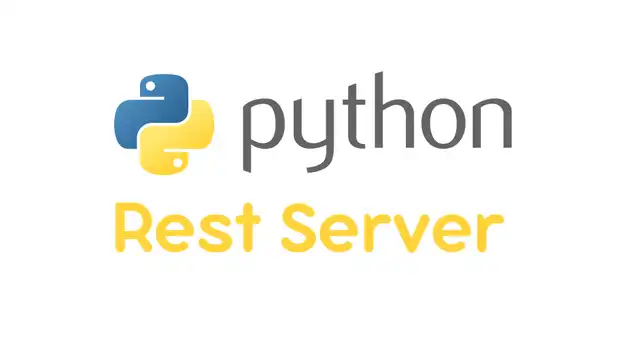
The benefits of using a REST Server are the following:
- REST servers are more efficient than traditional web servers because they use less CPU and memory.
- REST servers can be deployed more easily than traditional web servers.
- REST servers can provide a more efficient way to manage resources.
- REST servers can provide a more efficient way to access data from a web service.
What is a typical architecture for a REST API?
The Python Rest Server Architecture should include the following:
- A web server that is configured to serve Python applications
- A Python interpreter and application server that can be used to create and serve Python applications
- A database that is used to store data used by the applications
- An authentication and authorization system that allows users to access the applications
- A monitoring and logging system that can be used to monitor and track the performance of the applications
What popular Python libraries can be used to create a REST API?
Some popular Python libraries that can be used to create a REST API are:
- Requests: is a library that allows you to make HTTP requests
- Flask: is a library that allows you to create web applications in Python
- JsonPath: is a library that allows you to extract data from JSON objects
- Botocore: is a library that provides an API for managing bots
- SQLAlchemy: is a library that provides an API for managing databases
How does a Python Rest Server work?
To make a Python Rest Server, you first need to install Flask and Gunicorn. Then create a file named rest.py in your project’s root directory and add the following code:
Flask import Flask from gunicorn import app, wsgiref def main (): application = Gunicorn () application. config [ ‘WSGIServer’ ] = wsgiref . WSGIRestServer ( bind address: ‘/srv/webapps/ROI-SAMPLE/wsgi-app’, port: 8000 ) if __name__ == “__main__”: main ()
The code above defines a function named main that will be called when the server is started. This function allows you to configure the server by setting up the bind address and port parameters.
The next line sets up an instance of the gunicorn web worker for processing requests on behalf of your Flask application.
Finally, we define two functions: one that creates an application object and another that checks if it is being used and starts the application if necessary If everything has gone well, running Python ./rest_server.py should start up your Python Rest Server on port 8000!
How to Create a Python Rest Server with Flask and Gunicorn?
This blog post will walk you through creating a Python Rest Server with Flask and Gunicorn. This project will serve static files from a directory on your local machine.
We will also use the wsgiref module to bind our URLs.
- Create a new project by going to https://www- McMahon .stanford .edu/~bryanmckinney /Flask – REST – Server and clicking on the “Create New Project” button.
- Once the project has been created, click on the “Open in Browser” button to open it in your web browser.
- On the first screen of the creation wizard, select “Python 2 or 3?” If you are using Python 3, select “Python 3″ and leave all other options as they are (we will use Python 2 throughout this tutorial).
- Next, fill out all of the necessary information for your new Flask Rest Server project: Name (should be descriptive), Author (Bryan McKinnon), License (GPLv3), and Programming Language(Python). Click on Finish to finish up!
- Now that our new Flask Rest Server project is ready, let’s import some required libraries: gunicorn , flask, wsgiref . We need these libraries to deploy our server onto an actual hosting environment.
Conclusion
This blog post discussed how to build a Python Rest Server. We covered the following sections: what a Python Rest Server is, how it works, and how we put the Flask and Gunicorn together. Hopefully, you’ve found this blog post helpful in understanding the basics of building a Python Rest Server. If you have any questions or comments, please leave them below!
Add Comment